We went over this in class, but here is some documentation on the steps to secure your API keys using environment variables.
The Problem
When first working with an API, it's often easiest to include your API credentials in your code.
It might look something like this:
# put your API credentials here (usually found on your API dashboard)
account_sid = "YOUR_ACCOUNT_SID"
auth_token = "YOUR_AUTH_TOKEN"
Exposing your API credentials in a Github repository isn't the best idea, and it's a horrible idea if the repository is public! A public Github repository is just that, public - anyone can access it. And then they can use your API credentials as they wish. It's like sharing your account password with the world. #bad
It's so bad, in fact, that many API services (Google, Amazon, Twilio, etc) scan public repositories for their API keys and, if they recognize one, they immediately revoke it (making it unusable). If you pushed your code to Github with an API key, you may have received an email explaining this.
But even if your repository is private, API credentials that are in your code are still exposed to more people than necessary - everyone with access to the repository.
The Solution
Note: the following solution is applicable for securing any API keys. The example is for Twilio, but it's good practice to apply to all credentials that should not be viewable in your repository's code.
So the solution is to "hide" our API keys by storing them in "environment variables" - variables stored on the "server environment" instead of in our code.
Step 1 - Replace this code...
# put your API credentials here (found on your Twilio dashboard)
twilio_account_sid = "YOUR_TWILIO_ACCOUNT_SID"
twilio_auth_token = "YOUR_TWILIO_AUTH_TOKEN"
...with this code, where TWILIO_ACCOUNT_SID
is whatever variable you want (it could be TACOS
); and same for TWILIO_AUTH_TOKEN
.
# put your API credentials here (found on your Twilio dashboard)
twilio_account_sid = ENV["TWILIO_ACCOUNT_SID"]
twilio_auth_token = ENV["TWILIO_AUTH_TOKEN"]
If you have not yet added your API credentials into your code, just note that this is how you will do so when needed and move on to the next step.
Step 2 - Configure the environment variables in Gitpod:
To do so, go to gitpod.io/workspaces and click on your avatar in the top right. You'll see a dropdown. Click "Environment Variables".
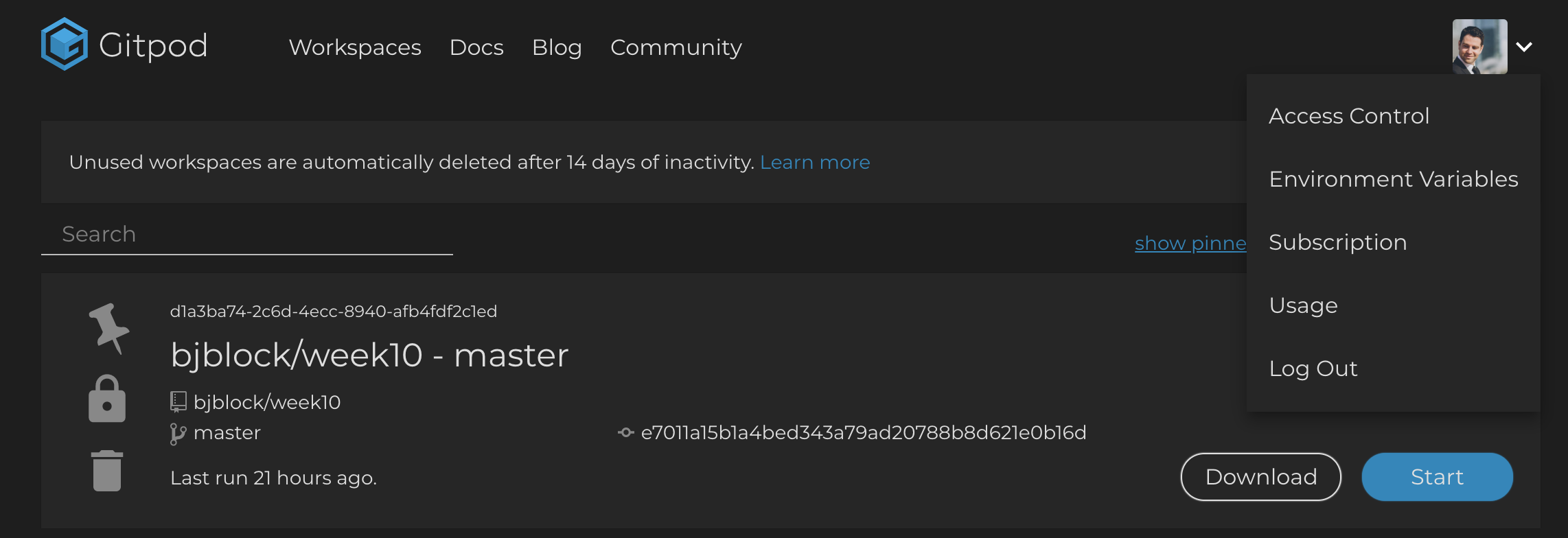
On the next screen, click "Add Variable". In the new row, the Name is the variable name you used above (e.g. TWILIO_ACCOUNT_SID
or TACOS
); the Value is the actual API key from Twilio; and the last column, the Organization/Repository needs to be */*
. Then repeat for the TWILIO_AUTH_TOKEN
. When you're done, it should look something like this:
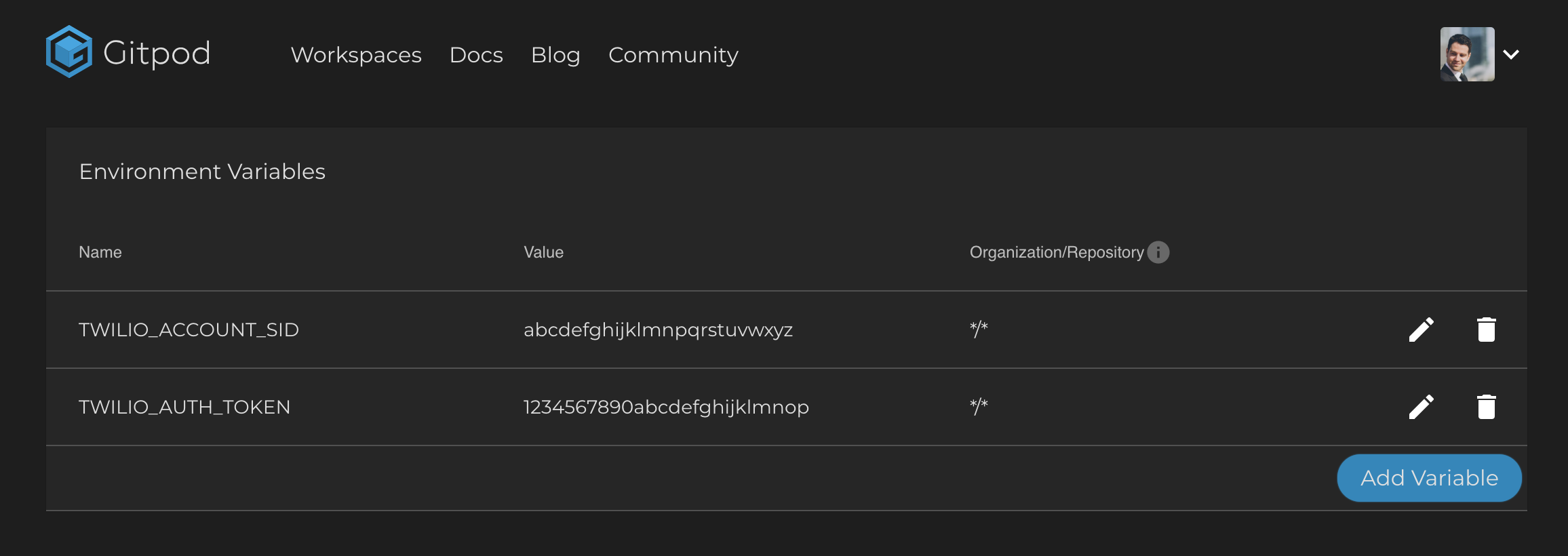
If you had your workspace running, you'll probably need to stop it and restart it so that your "environment" (i.e. workspace) recognizes these new environment variables. But then it should work properly.
Step 3 - Configure the environment variables in Heroku (optional):
If you're deploying your code to Heroku, you'll need to configure the environment variables there as well. Every "environment" needs to know about these variables.
Go to your Heroku dashboard and click on your application. Then navigate to the Settings tab. In the 2nd section, there's click "Reveal Config Vars". You'll see a list of environment variables that are preset by Heroku to make your application work in production. There should be an empty row at the bottom. Fill it in with the same Name and Value that you entered in Gitpod and click "Add". Repeat so that both Twilio API credentials are configured. It should look something like this when you're done:
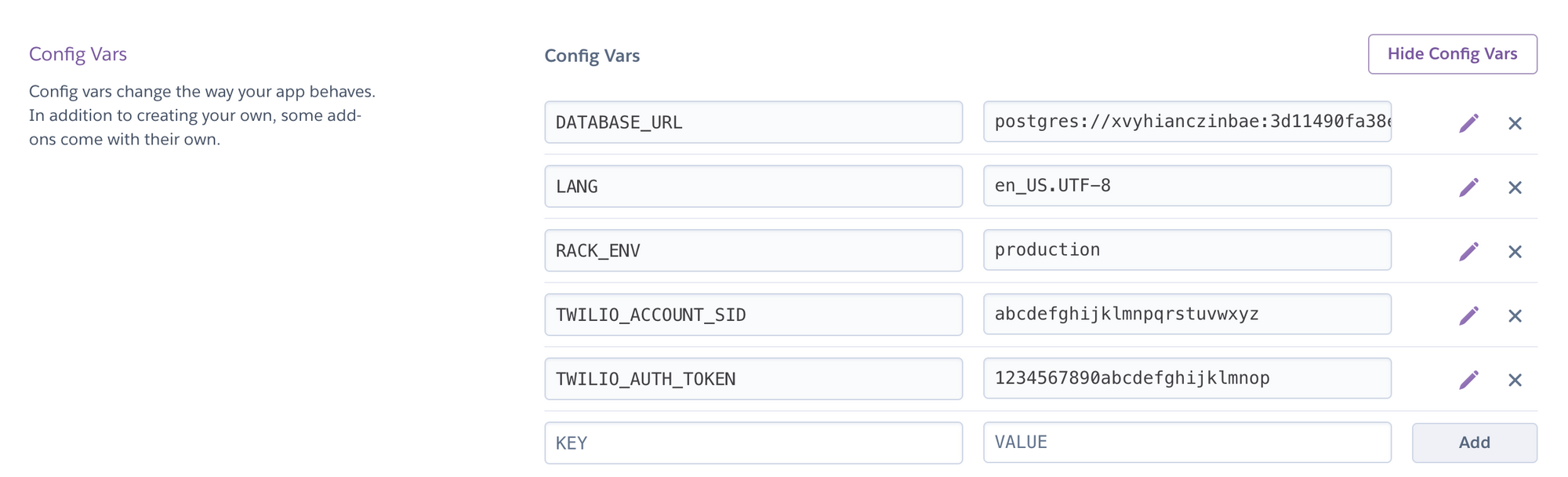
And that's it!